Audio System¶
Adding audio to your game is as easy as giving a drumstick to a rock star: they’ll know what to do. Lemonate supports two types of audio setups to cater to different game needs:
Simple Audio: This doesn’t care about object positions or cameras. Perfect for fixed-camera games or situations where sound sources stay constant.
Positional Audio: This makes sounds “spatial” by considering the location of sound sources and the player (or camera). Essential for immersive experiences like first-person shooters.
Simple Audio¶
Simple audio is ideal when you just want sound to “work” without worrying about who’s standing where. Background music, win fanfares, or interface sounds are perfect candidates.
Setting It Up¶
Go to the MainScene in the Inspector.
Add an AudioListener item (the game’s “ears”) and a few Audio items (the sounds).
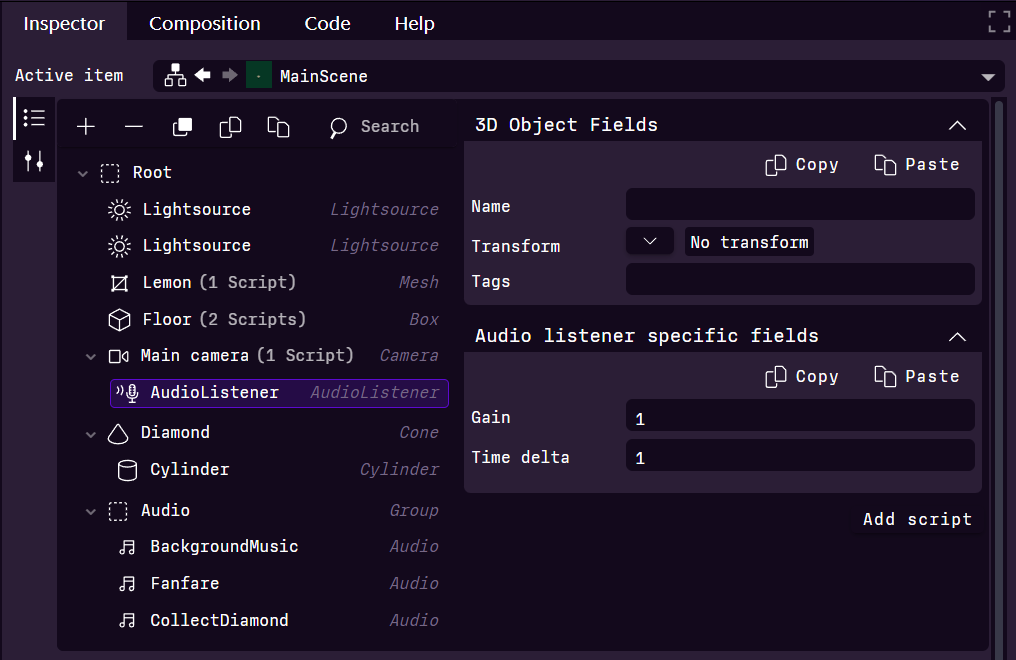
Example for simple audio setup¶
Assign audio assets to the Audio items. If you’re just testing, use audio assets from the asset store. For something more personal, you can import your own audio files later.
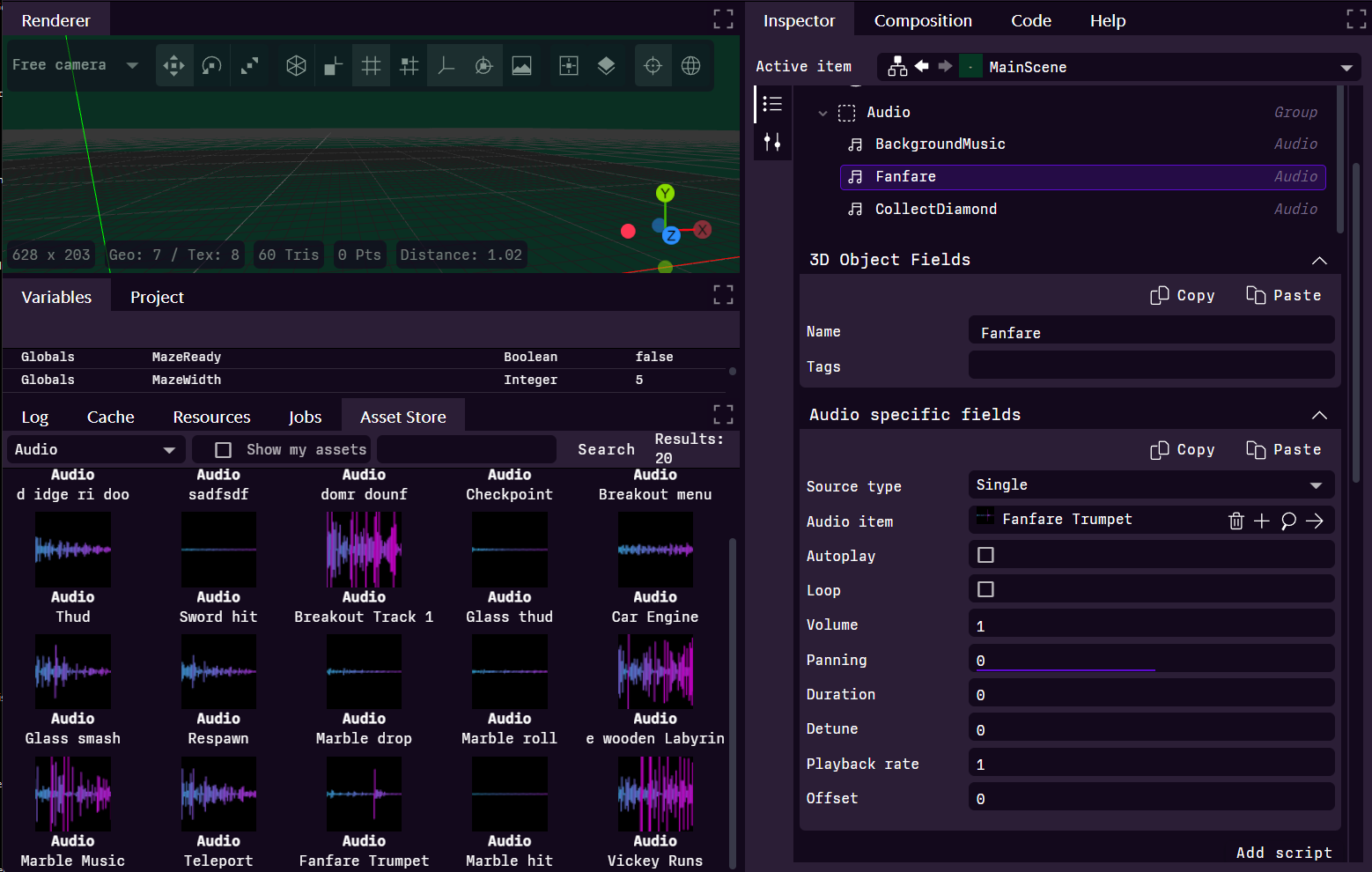
Link an audio asset to the audio item¶
Configure the sound parameters. For example: - Adjust the volume. - Enable Loop for background music (because silence in a maze is scarier than the maze itself).
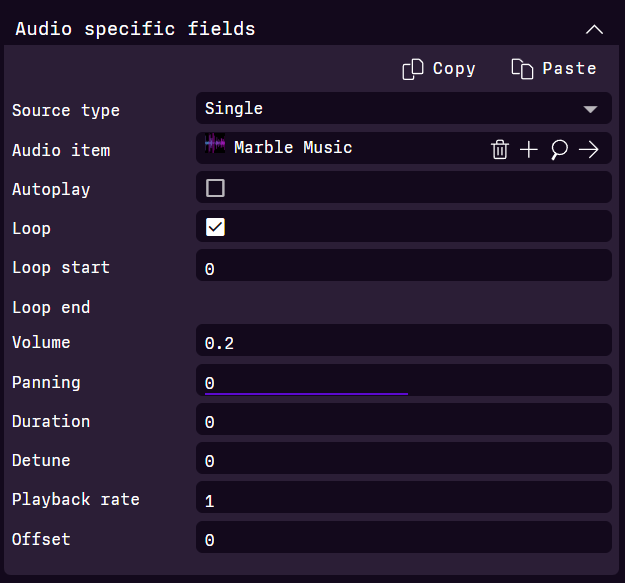
Set parameters for the audio items¶
Scripting Simple Audio¶
Now for the fun part—playing those sweet sounds in your scripts.
You can load and play audio items using the SceneObject:findByName(*name*) function. Here’s an example:
local backgroundMusic
local solvedMazeSound
local collectDiamondSound
function MazeController:init()
-- Load audio items by name
backgroundMusic = SceneObject:findByName("BackgroundMusic")
solvedMazeSound = SceneObject:findByName("Fanfare")
collectDiamondSound = SceneObject:findByName("CollectDiamond")
-- Start the background music
backgroundMusic:play()
end
function MazeController:update()
-- Check for game conditions and play sounds
if py > mazeSizeY + 1 then
print("Maze solved!")
solvedMazeSound:play()
end
end
Positional Audio¶
Positional audio makes your game sound more realistic by factoring in where the sounds come from relative to the player. Imagine hearing footsteps sneaking up behind you—spooky, right?
Where to Place the AudioListener?¶
Think of the AudioListener as the “ears” of your game. Its position determines where the player perceives sounds.
For first-person games, attach the AudioListener to the player camera—because, well, ears are usually close to eyes.
For third-person games, you can attach the AudioListener to the player character or the camera, depending on what makes sense for your game.
Setting Up Positional Audio¶
Replace the basic Audio items with PositionalAudio items. These items add 3D spatial effects to the sound.
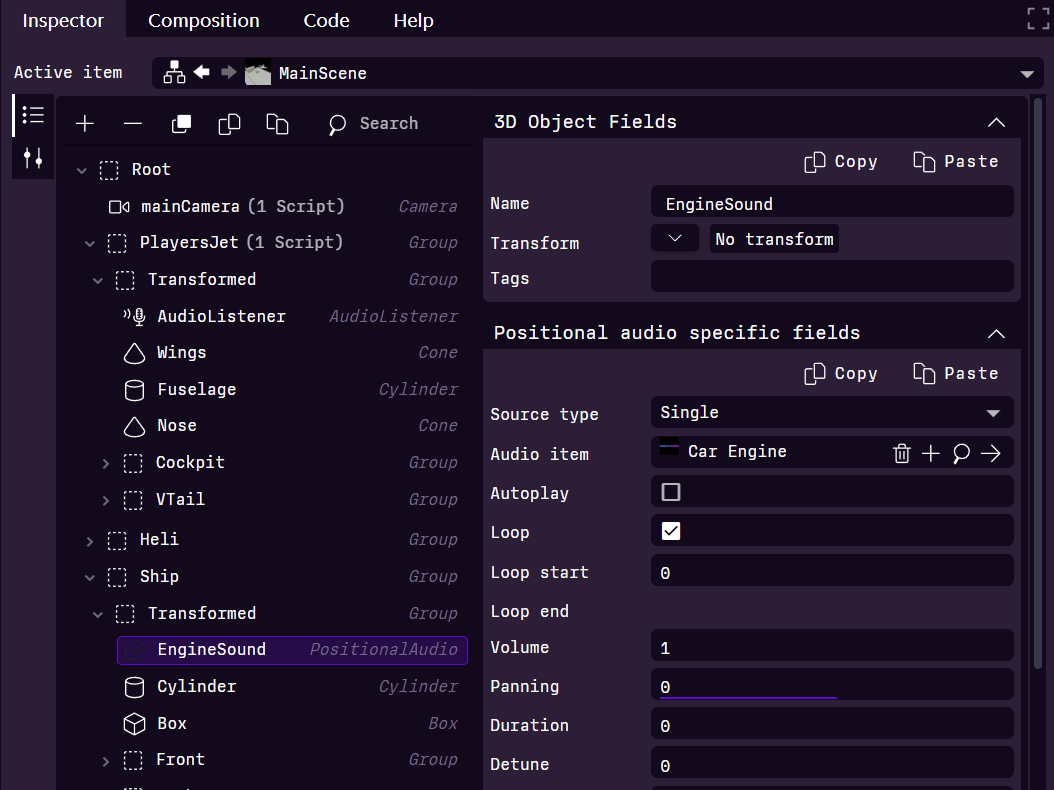
Example of the use of the PositionalAudio item¶
Add PositionalAudio items to the relevant game objects (e.g., a torch that crackles or an enemy robot that beeps ominously).
Place the AudioListener at the right spot—usually the player camera or character.
Extra Tips for Positional Audio¶
Directionality: If your sound comes from a specific direction (like a roaring dragon), test its positioning to ensure it “feels right.”
Testing: Use headphones! Spatial audio is best appreciated when you can hear sounds moving around you.
Summary¶
Adding sound is simple—making it immersive is the fun part. Use Simple Audio for straightforward, non-positional sounds, and switch to PositionalAudio for a spatial, immersive experience. With Lemonate’s audio system, your game can sound just as good as it looks—or better.