Lifecycle Methods¶
In our game engine, scripts written in Lua have access to specific lifecycle methods. These methods are automatically called by the engine to manage the behavior of scripts throughout the lifecycle of game objects. Understanding these methods is crucial for effectively controlling game logic, rendering sequences, and resource management.
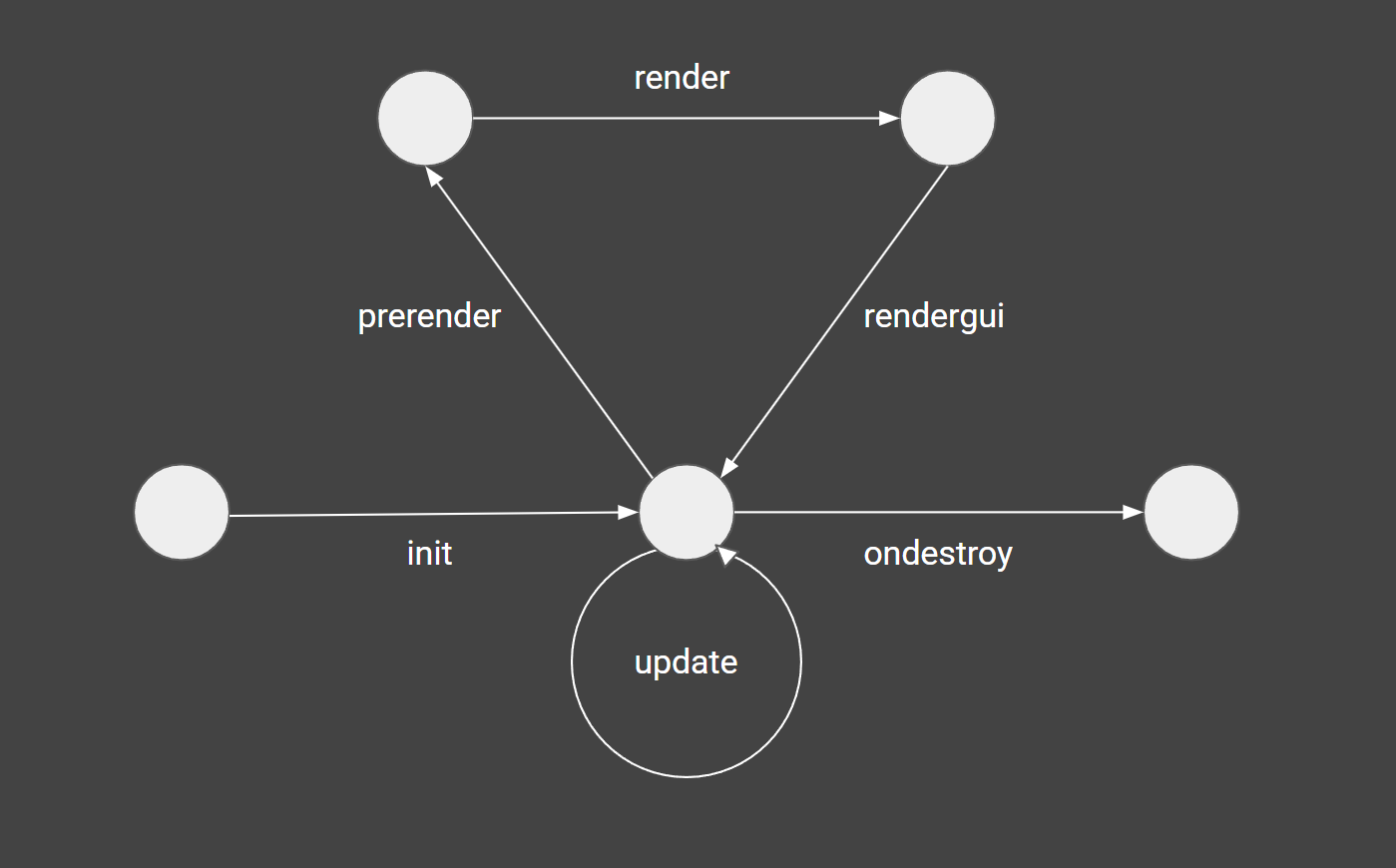
Lifecycle flow chart¶
Overview of Lifecycle Methods¶
Here are the primary lifecycle methods that your scripts can implement:
init()
update()
prerender()
render()
rendergui()
onenable()
ondisable()
ondestroy()
onpause()
onresume()
Below, we detail each method, its purpose, and its typical use cases.
init()¶
This method is called when a script is initially loaded and is generally used to set up initial state, bind events, or initialize variables. It is executed only once per script instance.
function MyEntity:init()
print("Script initialized")
-- Initialization code here
end
update()¶
The update() method is called repeatedly and is designed to handle the bulk of your game logic such as movement, input handling, and other frame-dependent operations. This method is called approximately 60 times per second. However, due to the single-threaded nature of the JavaScript-based engine, exact timing cannot be guaranteed; the actual rate may fluctuate based on frame rendering times. As can be seen in the flow chart, the update() cycle runs independently from the render cycle.
function MyEntity:update()
-- Code to update game state
end
prerender()¶
Called before the rendering process begins in each frame. Use this to update any states that need to be considered before the game renders the frame.
function MyEntity:prerender()
-- Pre-render logic here
end
render()¶
This method allows you to implement custom rendering code that executes during the scene’s render pass. It’s useful for drawing custom visuals or for making last-minute changes to the render state.
function MyEntity:render()
-- Custom rendering code
end
rendergui()¶
This method is specifically called to render GUI elements using ImGui functions. It is ideal for creating overlays, menus, and interactive GUI components within the game.
function MyEntity:rendergui()
-- GUI rendering code using ImGui
end
onenable()¶
Executed when the script is enabled. This is useful for re-initializing state when objects become active again.
function MyEntity:onenable()
print("Script enabled")
-- Activation code here
end
ondisable()¶
Called when the script is disabled. Use this to clean up or save state temporarily when objects or components are deactivated.
function MyEntity:ondisable()
print("Script disabled")
-- Cleanup code here
end
ondestroy()¶
This method is invoked just before the script’s instance is destroyed. It is used for important cleanup activities, such as releasing resources, saving state, or properly detaching events.
function MyEntity:ondestroy()
print("Script is being destroyed")
-- Cleanup code here
end
onpause()¶
This method is invoked if the game is paused with the editor or player’s pause button. It can be used to remember the timestamp and later do any kind of time adjustments in the oncontinue() function to make sure the game does not break due to this, like the player going to a timeout game over due to the pause.
function MyEntity:onpause()
print("Game is paused")
end
onresume()¶
This method is invoked if the game is resumed again
function MyEntity:onresume()
print("Game is resumed")
end
Best Practices¶
Performance Considerations: Since update() is called frequently, ensure that the code within is optimized and does not contain heavy computations.
Resource Management: Utilize ondestroy() to manage and release resources that are no longer required by the script.
State Management: Use onenable() and ondisable() to manage changes in script behavior when game objects are activated or deactivated.
Understanding and properly implementing these lifecycle methods will help you effectively manage your game logic and rendering tasks within the engine.