Variable Sets¶
Variable sets allow for the definition of globally accessible variables on the level of the project that can be used for storing things like number of lives, health, enemy counts and anything that needs to be accessed from more than just one script. Variable sets can also be reused between projects. Like many other things in Lemonate, they are an item and so can be packaged and reused. A project can contain multiple variable sets with a name and scripts can read and write to them using their variable set name and containing variable name. Variable sets can also be viewed in the Variables view in the Studio including their values.
Creating a variable set¶
Open up the inspector and jump to the project item. You will see a variable sets area. Add one entry, then create a new item in the variable set field and then give the new variable set an optional name. If you don’t give it a name, it will be available under the name of the item.
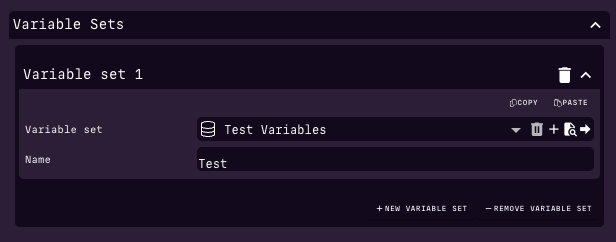
Then jump to the variable set item with the little right arrow at the end of the field. This will lead you to to the variable set item where you can create variables, give them a name, type and initial value. This is all you need to do to make them available to your game.
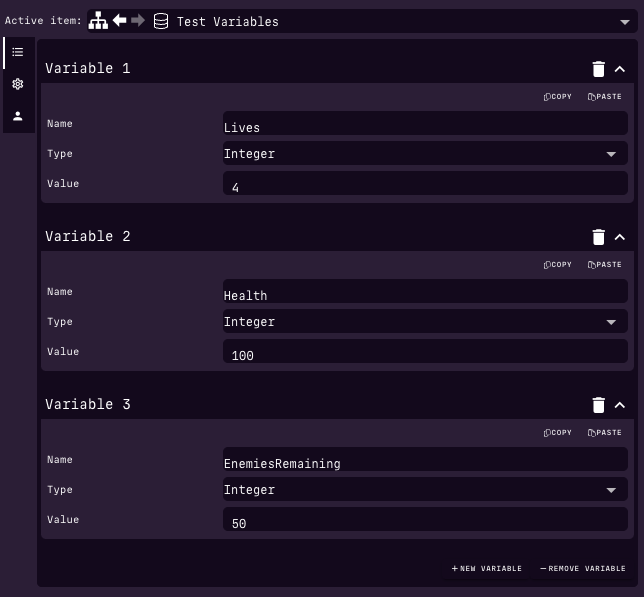
Check the variables view in the studio. The variables you created should have appeared. During playback this view will update so you can keep track of any changes to your variable values. This also makes them useful for debugging.
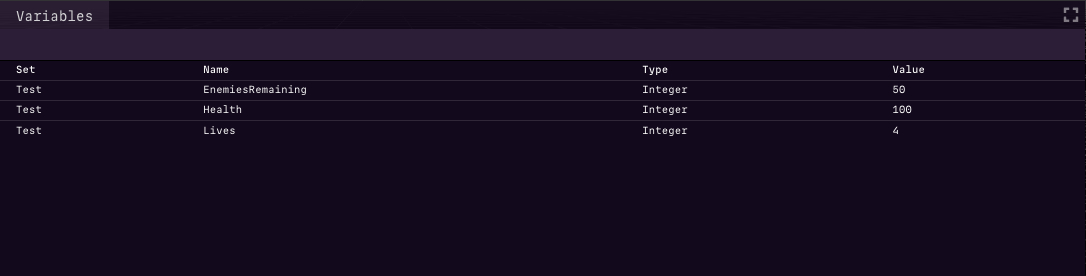
Using variables from code¶
The following script shows how to access variables by code. The functionality is available from the engine/variables module. See more information in the reference: Variables
local Variables = require 'engine/variables'
local Canvas = require 'engine/canvas'
local MyEntity = Class.new(Entity)
function MyEntity:init()
Variables.set("Test", "Set", 1)
Variables.add("Test", "Added", 4)
end
function MyEntity:render()
local set = Variables.get("Test", "Set")
local initial = Variables.get("Test", "Initial")
local added = Variables.get("Test", "Added")
Canvas.setFillColor(255, 255, 255, 1)
Canvas.setFont("20px Arial")
Canvas.fillText("Initial: " .. tostring(initial), 20, 40)
Canvas.fillText("Set: " .. tostring(set), 20, 60)
Canvas.fillText("Added: " .. tostring(added), 20, 80)
end
return MyEntity